2 min to read
Circuit Breaking in Distributed Systems
Enhancing Resilience with Circuit Breaker Patterns
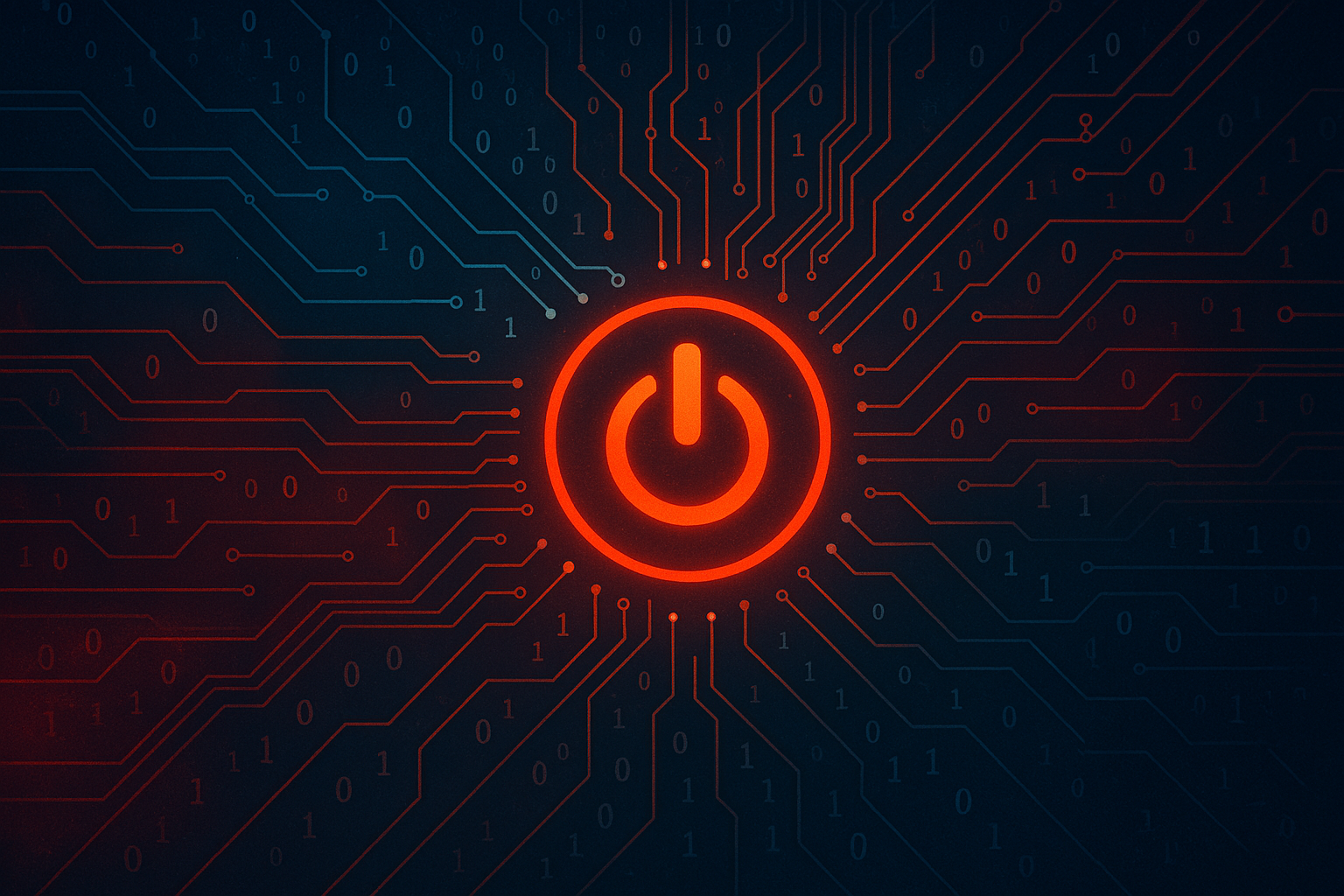
In modern distributed systems, failures are inevitable. The circuit breaker pattern helps maintain system stability by preventing failures from cascading across service boundaries. In this post, we’ll dive deep into circuit breakers, explore their importance, and demonstrate practical implementation using Resilience4j in Java.
Understanding the Circuit Breaker Pattern
The circuit breaker pattern prevents a service from making repeated calls to an external service that is experiencing issues. It has three main states:
- Closed: All requests pass through normally.
- Open: Requests fail fast, bypassing the problematic service.
- Half-Open: A limited number of requests test if the external service has recovered.
This mechanism helps improve the resilience and responsiveness of your systems.
When to Use Circuit Breakers
Circuit breakers are essential when:
- Your system relies on remote calls (microservices architecture, external APIs).
- You need to prevent failure propagation.
- You aim to improve user experience by quickly responding to failures.
Implementing Circuit Breakers with Resilience4j
Step 1: Adding Dependencies
Add Resilience4j dependencies to your project (Maven example):
<dependency>
<groupId>io.github.resilience4j</groupId>
<artifactId>resilience4j-circuitbreaker</artifactId>
<version>2.0.2</version>
</dependency>
Step 2: Configuring Circuit Breaker
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.permittedNumberOfCallsInHalfOpenState(2)
.minimumNumberOfCalls(5)
.build();
CircuitBreaker circuitBreaker = CircuitBreaker.of("myService", config);
Step 3: Using Circuit Breaker
Wrap service calls using the circuit breaker:
Supplier<String> decoratedSupplier = CircuitBreaker.decorateSupplier(circuitBreaker, () -> myService.remoteCall());
Try.ofSupplier(decoratedSupplier)
.recover(throwable -> "Fallback response")
.onSuccess(result -> System.out.println("Response: " + result));
Monitoring and Metrics
Resilience4j provides built-in support for metrics and monitoring:
CircuitBreakerRegistry registry = CircuitBreakerRegistry.ofDefaults();
registry.getEventPublisher()
.onStateTransition(event -> System.out.println(event.getStateTransition()));
Integrate with Prometheus, Grafana, or other observability tools for comprehensive monitoring.
Best Practices for Circuit Breaking
- Configure sensible thresholds to avoid premature circuit opening.
- Always define fallback mechanisms.
- Regularly monitor circuit breaker states and metrics.
- Test thoroughly to ensure reliability under failure conditions.
Conclusion
Implementing circuit breakers using Resilience4j significantly enhances the resilience of your Java-based distributed systems. This proactive approach ensures your systems gracefully handle failures, maintaining service availability and user satisfaction.