4 min to read
Mastering Concurrency in Java: Threads, Executors, and Virtual Threads
A Deep Dive into Java’s Multithreading and Parallel Computing
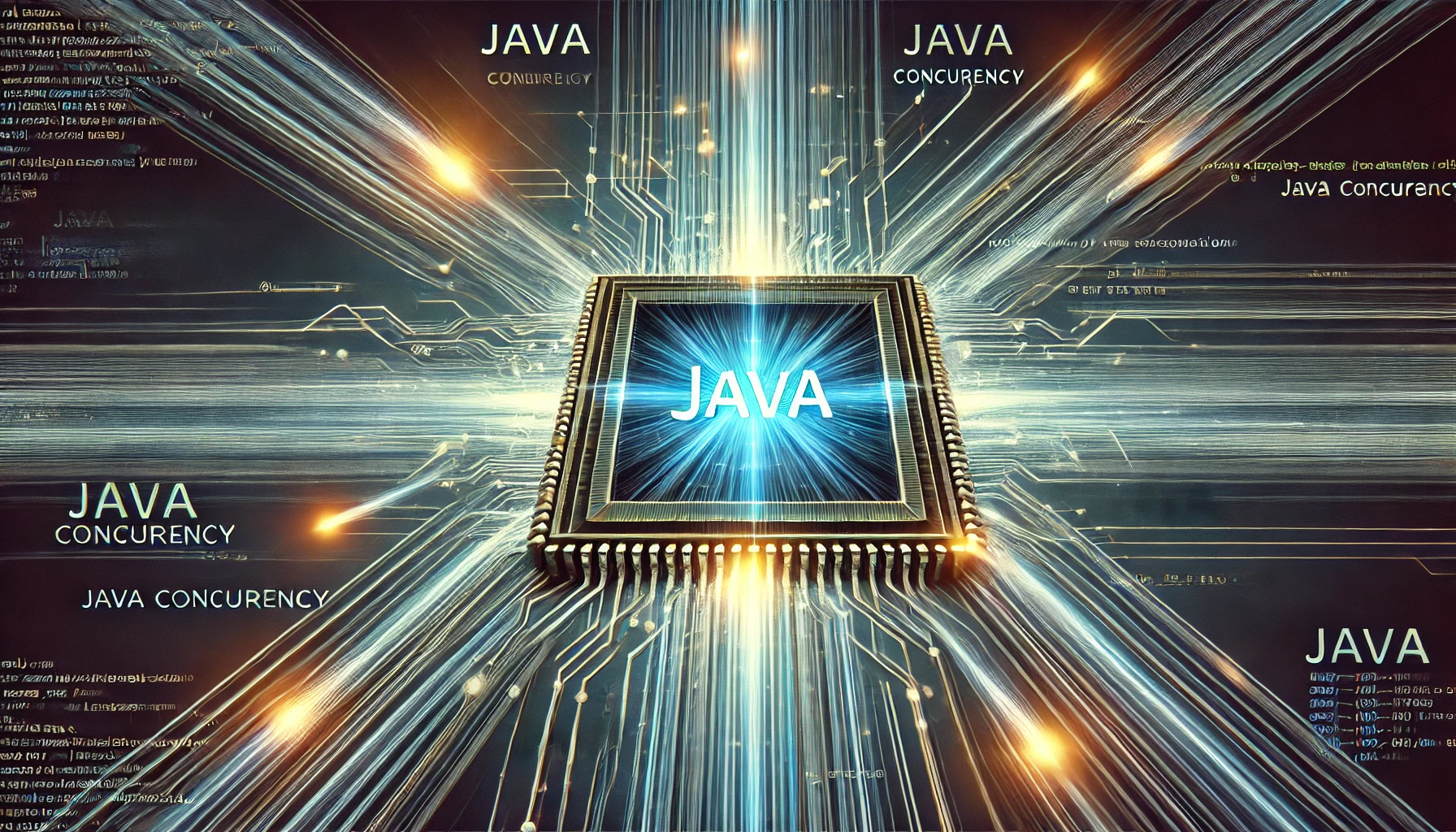
Java’s concurrency model is a critical component for building scalable and efficient applications. From classic Thread management to modern ExecutorService and the revolutionary Virtual Threads in Project Loom, mastering Java’s concurrency tools is essential for high-performance development.
Understanding Java’s Concurrency Model
Concurrency in Java enables applications to perform multiple tasks simultaneously, improving throughput and responsiveness. Java provides several concurrency primitives:
- Threads: The fundamental unit of execution, allowing tasks to run in parallel.
- Executors: High-level abstraction for managing thread pools and task execution.
- Virtual Threads: Lightweight, memory-efficient threads introduced in Java 21 for massive scalability.
- Fork/Join Framework: Designed for divide-and-conquer algorithms using parallel task execution.
- Reactive Programming: Asynchronous, non-blocking programming using event streams.
Let’s now take a deeper look at three major building blocks of concurrent programming in Java: classic threads, the ExecutorService
, and the new virtual threads.
Classic Approach: Java Threads
The most basic unit of concurrency in Java is the Thread
. You can extend the Thread
class or implement the Runnable
interface to define concurrent tasks.
class SimpleThreadExample extends Thread {
public void run() {
System.out.println("Thread running: " + Thread.currentThread().getName());
}
}
public class ThreadDemo {
public static void main(String[] args) {
SimpleThreadExample thread = new SimpleThreadExample();
thread.start();
}
}
Limitations of Raw Threads
- Performance Overhead: Creating and destroying threads is expensive.
- No Pooling: Each thread is a one-off. No built-in reuse.
- Scalability Issues: System resources limit the number of threads you can create.
Using raw threads for large-scale concurrent applications quickly becomes impractical.
Modern Concurrency: ExecutorService and Thread Pooling
To solve the limitations of raw threads, Java introduced the ExecutorService
as part of java.util.concurrent
. It provides a high-level API to manage and reuse threads through pooling.
How Thread Pooling Works
Thread pooling allows you to reuse a fixed number of threads to execute multiple tasks. Instead of creating a new thread for each task, the executor assigns tasks to available worker threads in the pool.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 0; i < 5; i++) {
executor.submit(() -> System.out.println("Task executed by: " + Thread.currentThread().getName()));
}
executor.shutdown();
}
}
Common Executor Types:
newFixedThreadPool(n)
: Fixed number of threads.newCachedThreadPool()
: Dynamically creates threads as needed.newSingleThreadExecutor()
: One thread for all tasks.newScheduledThreadPool(n)
: Supports delayed and periodic tasks.
Advantages:
- Efficient resource use through reuse.
- Better scalability and responsiveness.
- Built-in management of task queues and error handling.
The Future: Virtual Threads and Project Loom
Java 21 introduces Virtual Threads, a lightweight and scalable alternative to platform threads. A virtual thread is managed by the Java runtime rather than the operating system, allowing millions of threads to coexist.
Key Features:
- Minimal Memory Footprint: Much lighter than traditional threads.
- Unblocked I/O: I/O operations don’t block the underlying platform thread.
- Massive Scalability: Suitable for applications requiring thousands or millions of concurrent tasks (e.g., web servers).
Example:
public class VirtualThreadExample {
public static void main(String[] args) {
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
System.out.println("Running in: " + Thread.currentThread().getName());
});
}
}
}
}
When to Use Virtual Threads:
- I/O-bound applications (e.g., database access, HTTP clients).
- High-concurrency workloads (e.g., messaging systems).
- When simplifying thread management is a priority.
Conclusion
Java offers a flexible and powerful concurrency model. Classic Threads provide a foundational understanding, ExecutorService enables efficient thread reuse and task management, and Virtual Threads open the door to a new era of scalable concurrency with minimal overhead.
Each model has its place depending on the problem at hand. Mastering them gives you the toolkit needed to build high-performance, concurrent applications.
Further Reading
- Java Concurrency API
- Project Loom (Virtual Threads)
- Fork/Join Framework
- Effective Java - Joshua Bloch
Deep understanding of Java’s concurrency tools can significantly improve your system’s performance, scalability, and maintainability.